前置条件,成功安装odb,odb-mysql全套软件(参考之前博文)
原始官方demo跑不起来
官方文档demo地址:https://www.codesynthesis.com/products/odb/doc/manual.xhtml#2
需要另外参考:odb访问mysql数据库(odb的简单用法1):https://blog.csdn.net/woaichanganba/article/details/79841356
但其实这个文章,写的也不完整,需要结合官方文档才能跑起来。
01,代码准备
第一段person.cxx
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94
| #include <string> #include <odb/core.hxx> using namespace std; #pragma db object no_id class person { public: friend class odb::access; public: unsigned long id; string name; string sex; unsigned short age; }; ``` 第二段main.cpp ``` #include <iostream> #include <odb/database.hxx> #include <odb/transaction.hxx> #include <odb/mysql/database.hxx> #include "person-odb.hxx" using namespace std; using namespace odb::core; int main (int argc, char* argv[]) { person aben, wenhao; aben.id = 1;aben.name = "aben";aben.sex = "man";aben.age = 23; wenhao.id = 2;wenhao.name = "wenhao";wenhao.sex = "man";wenhao.age = 22; typedef odb::query<person> query; typedef odb::result<person> result; try { shared_ptr<odb::mysql::database> db; db.reset(new odb::mysql::database ("root", "123456", "test")) transaction t (db->begin ()); t.tracer (stderr_tracer); db->persist (aben); db->persist (wenhao); query q(query::id == 2); result r = db->query<person>(q); for (auto i:r) { cout << "{id:" << i.id << " name:" << i.name << " sex:" << i.sex << " age:" << i.age << "}" << endl; } t.commit (); } catch (const odb::exception& e) { cerr << e.what () << endl; } return 0; } ``` ## 02,生成person-odb.cxx,person-odb.hxx,person-odb.ixx ,person.sql。 命令:odb -d mysql --generate-query --generate-schema person.hxx 如果是sqlite需要增加参数--schema-format sql,否则生成的创建语句会集成到cxx代码中 sqlite版命令:odb -d sqlite --generate-query --generate-schema -schema-format sql person.hxx
 ## 03,生成数据表 mysql -u root -p create database odb_test 数据表导入数据库odb_test mysql --user=root --password=xxxxx --database=odb_test < person.sql ## 04,编译和运行 main.cpp中写死了用户名密码,需要做修改 g++ -std=c++11 -o driver main.cpp person-odb.cxx -lodb-mysql -lodb ./driver 
## 05,样例只有insert和select,update,delete参考 C++版本 ORM 访问数据库之ODB 的oracle Demo测试(二):https://www.cnblogs.com/hul201610101100/p/9482605.html ## 06,建表相关注意事项 表字段创建逻辑和坑 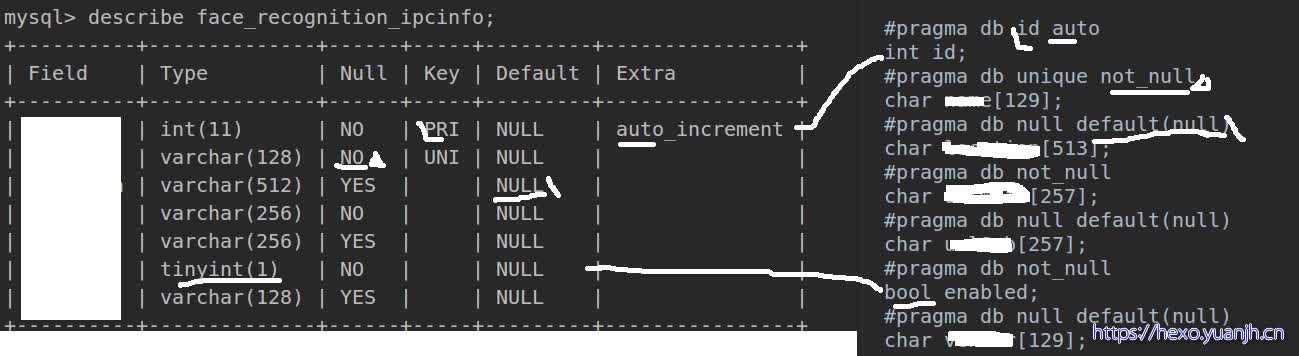
注意:
|
01,如果数据库字段varchar长度128,则对应cpp里长度+1=129,
02,int=>int(11)
03,auto=>auto_ioncrement
04,id=>primary key
05,unqiue=>unique key
06,not null => not null
07,default(null)=>default = null(需要在构造方法中设置字符串[0]=’\0’,避免出现随机值)
08,bool=>tinyint(1)
1 2 3 4 5 6 7 8 9 10
| 相关参考文档: 字段类型映射:https://www.codesynthesis.com/products/odb/doc/manual.xhtml#17.1 字段限制修饰符:https://www.codesynthesis.com/products/odb/doc/manual.xhtml#14.4 增删改查语句参考:https://www.codesynthesis.com/products/odb/doc/manual.xhtml#2.5 查询语句参考文档:https://www.codesynthesis.com/products/odb/doc/manual.xhtml#4.1 odb参数详情:https://www.codesynthesis.com/products/odb/doc/odb.xhtml
## 07,其他case readme:https://git.codesynthesis.com/cgit/odb/odb-examples/tree/hello/README
|
odb -d mysql –generate-query –generate-schema person.hxx
c++ -c person-odb.cxx
c++ -DDATABASE_MYSQL -c driver.cxx
c++ -o driver driver.o person-odb.o -lodb-mysql -lodb
mysql –user=root –password=xxxx –database=odb_test < person.sql
./driver –user root –password xxxx –database odb_test